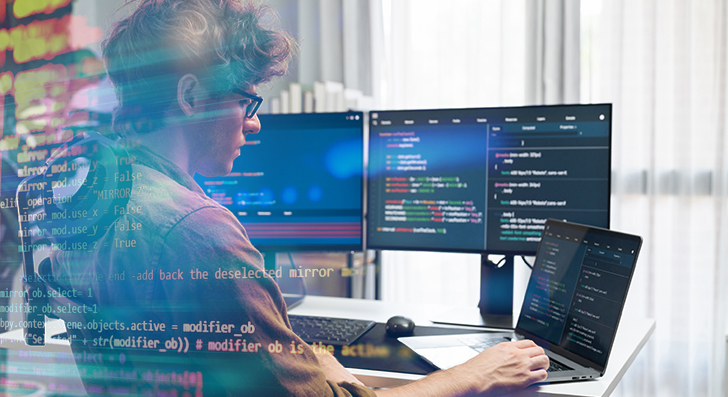
Scalability implies your software can take care of progress—much more users, additional knowledge, and a lot more site visitors—with out breaking. As a developer, developing with scalability in your mind saves time and stress afterwards. Right here’s a transparent and functional manual to assist you to start by Gustavo Woltmann.
Style and design for Scalability from the Start
Scalability just isn't one thing you bolt on afterwards—it should be section of the plan from the start. Quite a few programs are unsuccessful after they mature quickly because the initial structure can’t manage the extra load. For a developer, you have to Assume early about how your program will behave stressed.
Begin by coming up with your architecture to become adaptable. Steer clear of monolithic codebases wherever every thing is tightly linked. As a substitute, use modular style or microservices. These designs crack your application into smaller sized, unbiased components. Every single module or company can scale on its own without having influencing The complete method.
Also, take into consideration your databases from day just one. Will it need to handle 1,000,000 end users or merely 100? Choose the correct sort—relational or NoSQL—based on how your information will expand. Prepare for sharding, indexing, and backups early, Even when you don’t have to have them yet.
An additional crucial level is in order to avoid hardcoding assumptions. Don’t write code that only functions below existing situations. Think of what would transpire If the person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design styles that guidance scaling, like concept queues or function-driven techniques. These aid your app tackle extra requests without the need of finding overloaded.
Any time you Make with scalability in mind, you're not just preparing for fulfillment—you might be cutting down future problems. A very well-planned method is easier to take care of, adapt, and improve. It’s greater to organize early than to rebuild later.
Use the proper Databases
Picking out the appropriate database is usually a critical Portion of developing scalable purposes. Not all databases are created the identical, and using the Incorrect you can sluggish you down or even bring about failures as your app grows.
Start by knowledge your info. Is it highly structured, like rows inside of a table? If Certainly, a relational database like PostgreSQL or MySQL is a good healthy. These are generally strong with associations, transactions, and consistency. Additionally they assist scaling methods like browse replicas, indexing, and partitioning to deal with extra site visitors and data.
When your data is much more adaptable—like user activity logs, merchandise catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured knowledge and can scale horizontally a lot more conveniently.
Also, think about your read and compose styles. Are you currently undertaking many reads with fewer writes? Use caching and browse replicas. Are you presently handling a weighty generate load? Investigate databases which can deal with substantial produce throughput, or even occasion-based mostly facts storage systems like Apache Kafka (for short-term info streams).
It’s also sensible to Believe forward. You may not need to have Highly developed scaling features now, but choosing a database that supports them implies you gained’t need to have to change later.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your info dependant upon your entry designs. And generally watch databases general performance when you develop.
In brief, the correct database is determined by your app’s structure, speed needs, and how you anticipate it to develop. Consider time to choose properly—it’ll conserve lots of difficulty later.
Improve Code and Queries
Rapid code is vital to scalability. As your app grows, each small hold off provides up. Improperly published code or unoptimized queries can decelerate efficiency and overload your method. That’s why it’s crucial to build economical logic from the beginning.
Commence by writing clean up, uncomplicated code. Stay clear of repeating logic and take away anything at all pointless. Don’t pick the most intricate Remedy if a simple just one operates. Keep your capabilities quick, focused, and straightforward to check. Use profiling resources to uncover bottlenecks—spots exactly where your code usually takes way too lengthy to operate or employs an excessive amount of memory.
Future, examine your database queries. These normally sluggish matters down a lot more than the code itself. Be sure each query only asks for the info you actually have to have. Stay away from Find *, which fetches all the things, and as an alternative find certain fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, Specifically throughout large tables.
In case you see the identical facts currently being asked for again and again, use caching. Keep the effects temporarily making use of instruments like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your databases operations any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Remember to examination with massive datasets. Code and queries that work good with one hundred information may possibly crash once they have to deal with 1 million.
In a nutshell, scalable apps are rapidly applications. Maintain your code restricted, your queries lean, and use caching when wanted. These techniques assistance your software continue to be sleek and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more consumers and even more site visitors. If almost everything goes by way of one particular server, it is going to speedily become a bottleneck. That’s in which load balancing and caching can be found in. These two resources assist keep your application speedy, secure, and scalable.
Load balancing spreads incoming targeted traffic throughout several servers. Rather than 1 server doing all the do the job, the load balancer routes users to distinctive servers dependant on availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the others. Applications like Nginx, HAProxy, or cloud-centered remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing facts quickly so it could be reused rapidly. When buyers ask for exactly the same information yet again—like a product web site or possibly a profile—you don’t have to fetch it within the database every time. You may serve it in the cache.
There's two frequent types of caching:
one. Server-side caching (like Redis or Memcached) merchants information in memory for rapid access.
two. Client-aspect caching (like browser caching or CDN caching) stores static documents close to the consumer.
Caching decreases databases load, improves pace, and makes your app extra productive.
Use caching for things which don’t alter generally. And always be sure your cache is updated when info does improve.
In brief, load balancing and caching are uncomplicated but highly effective tools. Collectively, they assist your application deal with additional users, remain rapid, and Get better from difficulties. If you propose to grow, you will need both equally.
Use Cloud and Container Tools
To construct scalable purposes, you need equipment that allow your application mature effortlessly. That’s in which cloud platforms and containers can be found in. They offer you adaptability, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and companies as you require them. You don’t should invest in components or guess future capacity. When visitors raises, you'll be able to incorporate far more assets with just a couple clicks or routinely working with car-scaling. When website traffic drops, you may scale down to economize.
These platforms also offer services like managed databases, storage, load balancing, and stability applications. You could deal with setting up your application as an alternative to controlling infrastructure.
Containers are Yet another important tool. A container offers your application and almost everything it has to run—code, libraries, configurations—into one device. This can make it effortless to move your application involving environments, from the laptop to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
When your application employs several containers, resources like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If just one portion of one's application crashes, it restarts it routinely.
Containers also allow it to be straightforward to independent parts of your application into solutions. You could update or scale areas independently, that is perfect for overall performance and trustworthiness.
In brief, using cloud and container equipment means you may scale rapidly, deploy easily, and Get well quickly when troubles happen. In order for you your app to increase without limitations, get started making use more info of these instruments early. They save time, lessen risk, and enable you to continue to be focused on creating, not correcting.
Monitor Anything
In the event you don’t keep an eye on your software, you won’t know when items go Mistaken. Checking helps you see how your app is undertaking, location problems early, and make greater conclusions as your application grows. It’s a key Portion of making scalable units.
Begin by tracking simple metrics like CPU use, memory, disk space, and response time. These let you know how your servers and companies are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you collect and visualize this information.
Don’t just check your servers—keep an eye on your application way too. Control how much time it's going to take for users to load pages, how often errors happen, and exactly where they happen. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical troubles. By way of example, When your response time goes over a limit or perhaps a service goes down, you should get notified immediately. This helps you take care of challenges rapid, typically ahead of consumers even discover.
Monitoring is usually handy if you make improvements. When you deploy a whole new function and find out a spike in glitches or slowdowns, it is possible to roll it back right before it will cause actual harm.
As your application grows, targeted traffic and info increase. Devoid of monitoring, you’ll pass up indications of difficulty right until it’s way too late. But with the proper applications in position, you continue to be in control.
In short, checking aids you keep the app reliable and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for big firms. Even small applications have to have a powerful Basis. By creating thoroughly, optimizing wisely, and using the ideal resources, you may Develop applications that develop efficiently without breaking under pressure. Start out little, Consider significant, and Construct clever.